IOException
In Java, IOException is one of the most common Exceptions when the program operates between input and output.
IOException is used for input and output-related operations. Sometimes it’s called Input-Output Exception.
Whatever the reason for IOException
- Reading from a file server but network disconnected,
- Read a file from the local machine but you do not have a file with that name,
- Streaming data but at that moment other processes run on the same port and stop the data stream,
- Want to read or write a file but have no permission,
- Want to write some data to a file but no disk space.
In such cases IOException occurs.
IOException is a checked exception, so programmer code will tell you the reasons for the exception, so that bugs or code errors can be easily fixed.
FileNotFoundException, EOFException,
UnsupportedEncodingException, SocketException, SSLException These are IOException er subclass.
⏭ If the file is not found, FileNotFoundException will occur.
⏭ SSLException will occur if there is no SSL connection.
⏭ EOFException will occur when you finish reading the file.
⏭ UnsupportedEncodingException occurs when unsupported files are encoded.
⏭ SocketException will occur when the socket connection is closed.
1.FileNotFoundException
I have created a method called checkFileNotFound which will check if the text.txt file exists or not.
public class IOExceptionExample {
public void checkFileNotFound(){
try {
FileInputStream inputStream = new FileInputStream("text.txt");
System.out.println("This is not Printed!");
}catch (FileNotFoundException e){
System.out.println(e);
}
}
public static void main(String args[]){
IOExceptionExample ioExceptionExample = new IOExceptionExample();
ioExceptionExample.checkFileNotFound();
}
}
output:
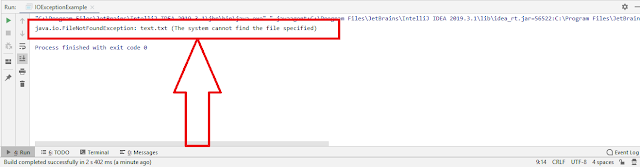
hope you got it!