Hello artisan,
How are you? Today I am going to show you how to upload images in your Laravel project. In this tutorial, you can also upload your image reference to your database also. That means you can upload your image to the Database and also you can fetch images from your directory using DB image name reference.
Our final Output looks like:
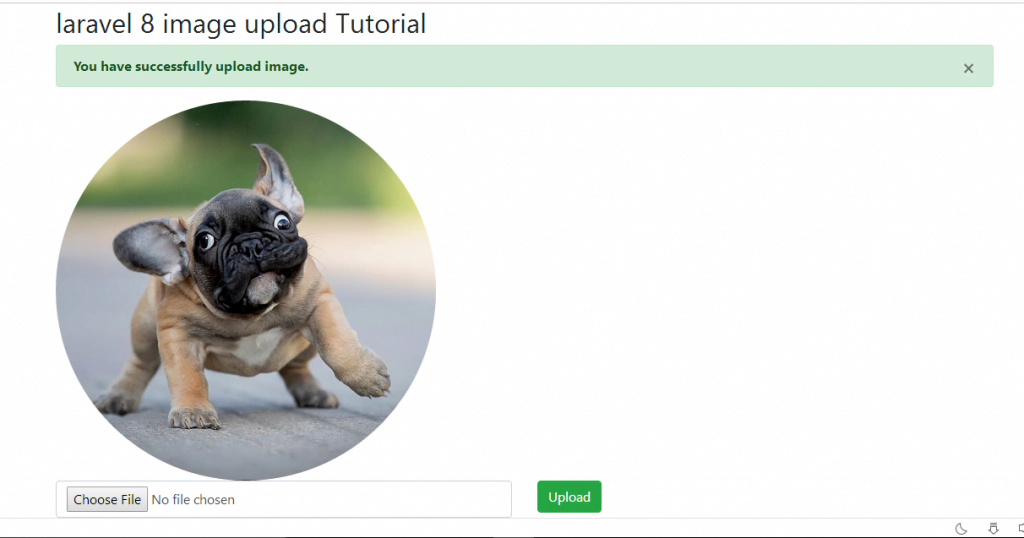
Create a Laravel Project first, run this command
composer create-project --prefer-dist laravel/laravel blog
Make databse Connection , create a database in the Mysql database after that go to the .env file and set the values,
Make Database Connection and add the code to .env file
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel_crud
DB_USERNAME=root
DB_PASSWORD=
Make your custom route,
routes/web.php
Route::get('/image/upload', [UploadImageController::class, 'imageUpload'])->name('image.upload.index');
Route::post('/image/upload/store', [UploadImageController::class, 'imageUploadPost'])->name('image.upload.store');
Create Controller
app/Http/controllers/UploadImageController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UploadImageController extends Controller
{
public function imageUpload(){
return view('image.image_upload');
}
public function imageUploadPost(Request $request){
$request->validate([
'image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048',
]);
$imageName = time().'.'.$request->image->extension();
//return $imageName;
$request->image->move(public_path('images'), $imageName);
/* Store $imageName name in DATABASE from HERE */
return back()
->with('success','You have successfully upload image.')
->with('image',$imageName);
}
}
Create View
resources/views/image_upload.blade.php
<!DOCTYPE html>
<html>
<head>
<title>laravel 8 image upload Tutorial</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css">
</head>
<body>
<div class="container mt-5">
<div class="panel panel-primary">
<div class="panel-heading"><h2>laravel 8 image upload Tutorial</h2></div>
<div class="panel-body">
@if ($message = Session::get('success'))
<div class="alert alert-success alert-block">
<button type="button" class="close" data-dismiss="alert">×</button>
<strong>{{ $message }}</strong>
</div>
<img style="width: 450px;height: 450px; border-radius: 50%" src="{{ URL::to('/') }}/images/{{ Session::get('image') }}">
@endif
@if (count($errors) > 0)
<div class="alert alert-danger">
<strong>Whoops!</strong> There were some problems with your input.
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
<form action="{{route('image.upload.store') }}" method="POST" enctype="multipart/form-data">
@csrf
<div class="row">
<div class="col-md-6">
<input type="file" name="image" class="form-control">
</div>
<div class="col-md-6">
<button type="submit" class="btn btn-success">Upload</button>
</div>
</div>
</form>
</div>
</div>
</div>
</body>
</html>
Output
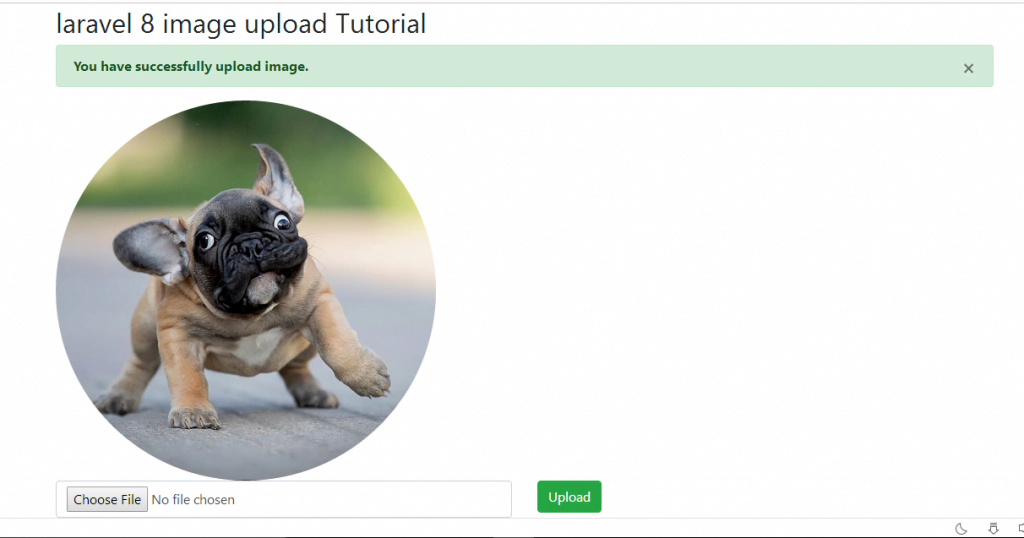