Hello, Today I am going to show you how to pass the client id and secret using the header. In Oauth2 there needs to be a pass the client id and secret to every request you made. In the header section, there might be some configuration you have to create to make any request. Sometimes you have to configure the response body like the content type which actually you want to fetch.
So, today I am going to show you how to send the client id and secret using the header.
Firstly you have to encode the client id and secret. So for this, go to this website and encode the client id and secret like me:
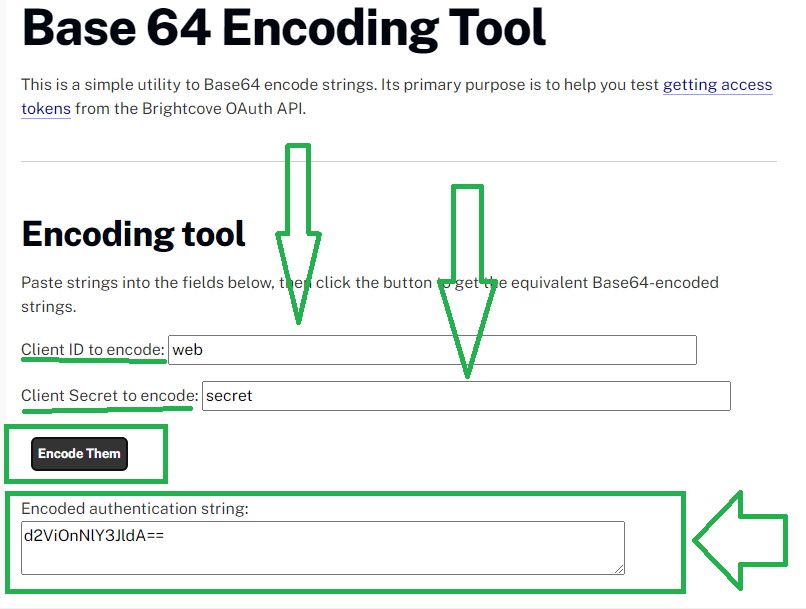
After encoded, configure the headers with “‘Authorization’: ‘Basic d2ViOnNlY3JldA==’, ” like that. Remember don’t forget to add the “Basic” before the encoded value in the first.
Axios Request Format
var axios = require('axios');
var data = JSON.stringify({"firstName":"Jone","lastName":"Doe","email":"[email protected]","mobile":"82492222d","password":"@password@"});
var config = {
method: 'post',
url: 'http://localhost:9191/api/v1/auth-service/customer/registration',
headers: {
'Authorization': 'Basic d2ViOnNlY3JldA==',
'Content-Type': 'application/json'
},
data : data
};
axios(config)
.then(function (response) {
console.log(JSON.stringify(response.data));
})
.catch(function (error) {
console.log(error);
});
In this way you can send the client Id and Secret using any http/https request header.
Javascript fetch Request Format
var myHeaders = new Headers();
myHeaders.append("Authorization", "Basic d2ViOnNlY3JldA==");
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({"firstName":"Jone","lastName":"Doe","email":"[email protected]","mobile":"866592222","password":"@password@"});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("http://localhost:9191/api/v1/auth-service/customer/registration", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
Javascript Jquery Format
var settings = {
"url": "http://localhost:9191/api/v1/auth-service/customer/registration",
"method": "POST",
"timeout": 0,
"headers": {
"Authorization": "Basic d2ViOnNlY3JldA==",
"Content-Type": "application/json"
},
"data": JSON.stringify({"firstName":"Jone","lastName":"Doe","email":"[email protected]","mobile":"866592222","password":"@password@"}),
};
$.ajax(settings).done(function (response) {
console.log(response);
});
Hope this might be helpful. If have any queries please feel free to comment.