Hello Artisan,
Today we will make form validation strategies to make input data validated.
Our final output looks like
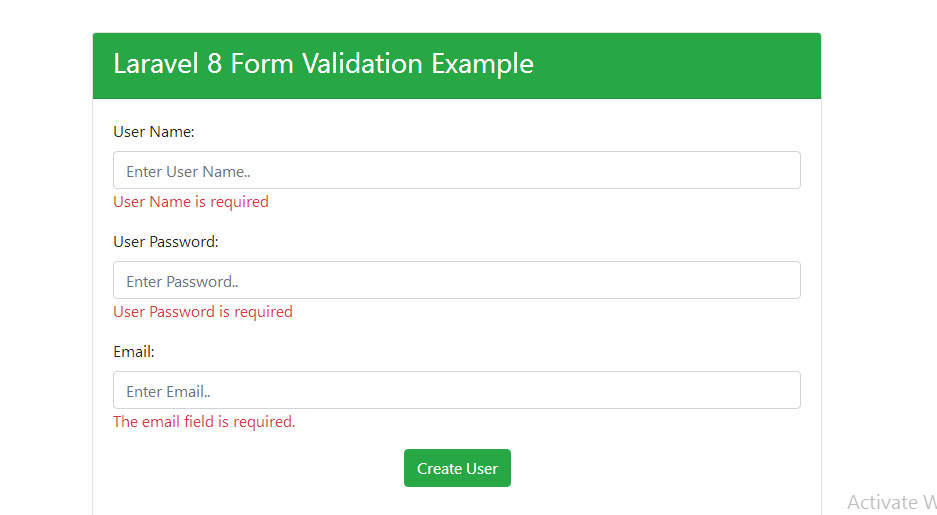
Create a Laravel Project first, run this command
composer create-project –prefer-dist laravel/laravel blog
Make databse Connection , create a database in the Mysql database after that go to the .env file and set the values,
Make Database Connection
and add the code to .env file
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel_blog
DB_USERNAME=root
DB_PASSWORD=
Create your custom route,
routes/web.php
Route::get('user/create',[UserController::class,'create']);
Route::post('user/store',[UserController::class,'store']);
After creating a brand new laravel project you have already the User model and UserController in your project directory. If not then add
Create Model
Models/User.php
<?php
namespace App\Models;
use Illuminate\Contracts\Auth\MustVerifyEmail;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
class User extends Authenticatable
{
use HasFactory, Notifiable;
/**
* The attributes that are mass assignable.
*
* @var array
*/
protected $fillable = [
'name', 'email', 'password',
];
/**
* The attributes that should be hidden for arrays.
*
* @var array
*/
protected $hidden = [
'password', 'remember_token',
];
/**
* The attributes that should be cast to native types.
*
* @var array
*/
protected $casts = [
'email_verified_at' => 'datetime',
];
}
Create Controller
app/Http/controllers/UserController.php
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function create(){
return view('user.create_user');
}
public function store(Request $request){
// dd($request);
$validatedData = $request->validate([
'name' => 'required',
'password' => 'required|min:5',
'email' => 'required|email|unique:users'
], [
'name.required' => 'User Name is required',
'password.required' => 'User Password is required'
]);
$validatedData['password'] = bcrypt($validatedData['password']);
$user = User::create($validatedData);
return back()->with('success', 'User created successfully.');
}
}
Also you have migration file, if not then add
database/migrations/2014_10_12_000000_create_users_table.php
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateUsersTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('users');
}
}
Run Migrate command to Create table to the database
php artisan migrate
Create your view
resources/views/user_create.blade.php
<!DOCTYPE html>
<html>
<head>
<title>Laravel 8 Form Validation Example - NiceSnippets.com</title>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha512-MoRNloxbStBcD8z3M/2BmnT+rg4IsMxPkXaGh2zD6LGNNFE80W3onsAhRcMAMrSoyWL9xD7Ert0men7vR8LUZg==" crossorigin="anonymous" />
</head>
<body>
<div class="container">
<div class="row mt-5">
<div class="col-8 offset-2 mt-5">
<div class="card">
<div class="card-header bg-success">
<h3 class="text-white">Laravel 8 Form Validation Example</h3>
</div>
<div class="card-body">
@if(Session::has('success'))
<div class="alert alert-success">
{{ Session::get('success') }}
@php
Session::forget('success');
@endphp
</div>
@endif
<form method="POST" action="{{ url('user/store') }}">
@csrf
<div class="form-group">
<label>User Name:</label>
<input
type="text"
name="name"
value="{{ old('name') }}"
class="form-control"
placeholder="Enter User Name..">
@if ($errors->has('name'))
<span class="text-danger">{{ $errors->first('name') }}</span>
@endif
</div>
<div class="form-group">
<label>User Password:</label>
<input
type="password"
name="password"
class="form-control"
placeholder="Enter Password..">
@if ($errors->has('password'))
<span class="text-danger">{{ $errors->first('password') }}</span>
@endif
</div>
<div class="form-group">
<label>Email:</label>
<input
type="text"
name="email"
value="{{ old('email') }}"
class="form-control"
placeholder="Enter Email..">
@if ($errors->has('email'))
<span class="text-danger">{{ $errors->first('email') }}</span>
@endif
</div>
<div class="form-group text-center">
<button class="btn btn-success btn-submit">Create User</button>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
Output:
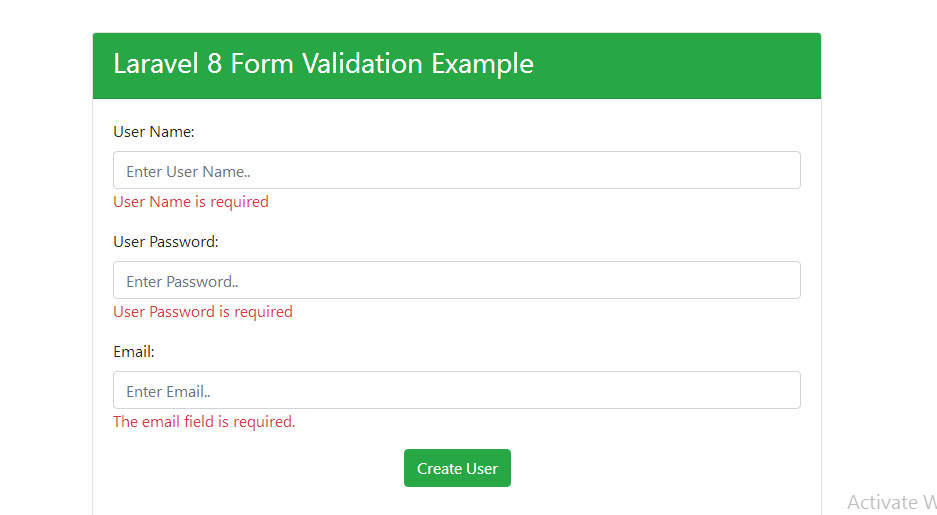
I think, it will help you!