Hello Artisan,
Today we will create a CRUD application in Laravel using Mysql Database. CRUD extends Create, Read, Update, Delete. We performing This operation in our new fresh laravel project. So, let’s start.
Our final output looks like
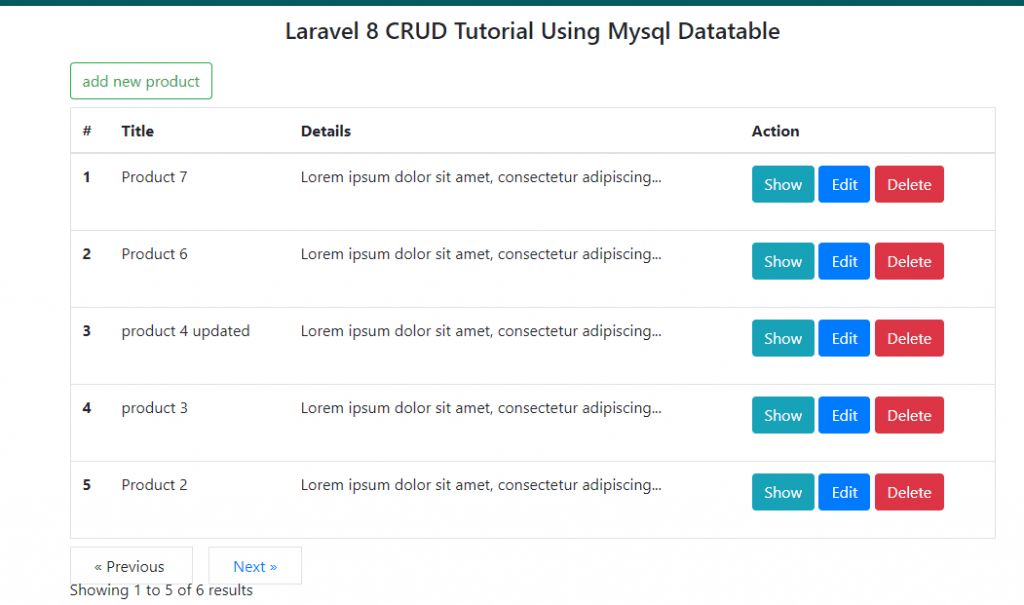
Create a Laravel Project first, run this command
composer create-project --prefer-dist laravel/laravel blog
After completion the creation of laravel project, lets go…
Make databse Connection , create a databse in the mysql database after that go to the .env file
and add the code
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel_crud
DB_USERNAME=root
DB_PASSWORD=
Set your Databasae name,username and password.
Now, run this command to migrate
php artisan migrate
Create Product model
php artisan make:model Product
Create migration for products table, run this command
php artisan make:migration create_products_table --create=products
let’s add products table column propertise to the migration file.
Schema::create('products', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('details');
$table->timestamps();
});
Create Controller, run this command
php artisan make:controller ProductController --resource
In web.php add our route,
web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\ProductController;
use App\Http\Controllers\UserController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function () {
return view('index');
});
Route::resource('product',ProductController::class);
To see out all route, run this command
php artisan route:list
Output
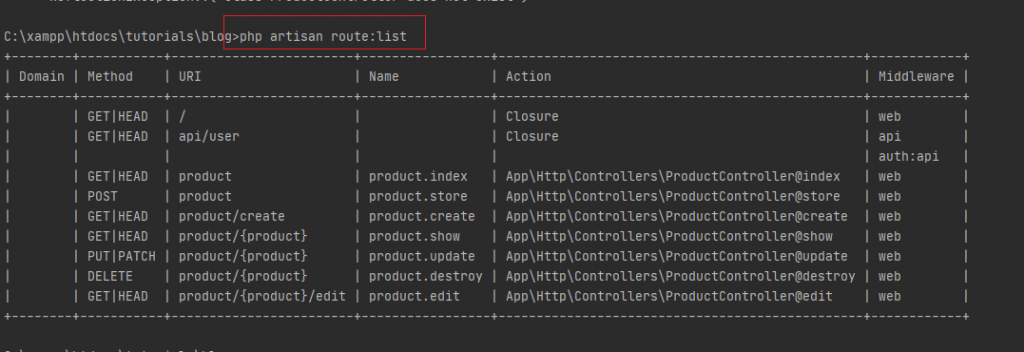
Models/Product.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Product extends Model
{
use HasFactory;
protected $fillable = [
'title',
'details'
];
}
controllers/ProductController.php
<?php
namespace App\Http\Controllers;
use App\Models\Product;
use Illuminate\Http\Request;
class ProductController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$products = Product::latest()->paginate(5);
return view('index',compact('products'))
->with('i', (request()->input('page', 1) - 1) * 5);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('add_product');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$request->validate([
'title' => 'required',
'details' => 'required',
]);
//dd($request);
//return $request->all();
Product::create($request->all());
return redirect()->route('product.index')
->with('success','Product added successfully.');
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show(Product $product)
{
return view('product_details',compact('product'));
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit(Product $product)
{
return view('edit_product',compact('product'));
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, Product $product)
{
$request->validate([
'title' => 'required',
'details' => 'required',
]);
$product->update($request->all());
return redirect()->route('product.index')
->with('success','Product updated successfully');
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy(Product $product)
{
$product->delete();
return redirect()->route('product.index')
->with('success','Product deleted successfully');
}
}
Let’s create our views,
resources/views/layout/app.blade.php
<html>
<head>
<title>Laravel 8 CRUD Tutorial Using Mysql Datatable</title>
<meta name="csrf-token" content="{{ csrf_token() }}">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha512-MoRNloxbStBcD8z3M/2BmnT+rg4IsMxPkXaGh2zD6LGNNFE80W3onsAhRcMAMrSoyWL9xD7Ert0men7vR8LUZg==" crossorigin="anonymous" />
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/js/bootstrap.min.js"></script>
</head>
<style type="text/css">
.container{
margin-top:10px;
}
h4{
margin-bottom:20px;
}
</style>
<body>
<div class="container">
@yield('main-content')
</div>
</body>
</html>
resourses/views/index.blade.php
@extends('layout.app')
@section('main-content')
<div class="row">
<div class="col-md-10 offset-md-2">
<div class="row">
<div class="col-md-12 text-center">
<h4>Laravel 8 CRUD Tutorial Using Mysql Datatable</h4>
</div>
<a href="{{route('product.create')}}" class=" btn btn-outline-success mb-2">add new product</a>
<div class="col-md-12">
@if ($message = Session::get('success'))
<div class="alert alert-success">
<p>{{ $message }}</p>
</div>
@endif
</div>
<table class="table border">
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Title</th>
<th scope="col">Details</th>
<th>Action</th>
</tr>
</thead>
<tbody>
@foreach($products as $product)
<tr>
<th scope="row">{{ ++$i}}</th>
<td>{{$product->title}}</td>
<td>{{\Str::limit($product->details, 50)}}</td>
<td>
<form action="{{ route('product.destroy',$product->id) }}" method="POST">
<a class="btn btn-info" href="{{ route('product.show',$product->id) }}">Show</a>
<a class="btn btn-primary" href="{{ route('product.edit',$product->id) }}">Edit</a>
@csrf
@method('DELETE')
<button type="submit" class="btn btn-danger">Delete</button>
</form>
</td>
</tr>
@endforeach
</tbody>
</table>
{!! $products->links() !!}
</div>
</div>
</div>
</div>
@endsection
add product view file
resourses/views/add_product.blade.php
@extends('layout.app')
@section('main-content')
<div class="row mt-1">
<div class="col-md-8 offset-md-2">
<div class="row">
<div class="col-md-12 text-center">
<h4>Laravel 8 CRUD Tutorial Using Mysql Datatable</h4>
</div>
<div class="col-md-12 mt-1 mr-1">
<div class="float-right">
<a class="btn btn-primary" href="{{ route('product.index') }}"> Back</a>
</div>
</div>
<div class="col-md-12">
@if ($errors->any())
<div class="alert alert-danger">
<strong>Whoops!</strong> Please input properly!!!<br><br>
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
</div>
<div class="col-md-12">
<form method="post" action="{{route('product.store')}}">
@csrf
<div class="form-group">
<label for="title">Title:</label>
<input
type="text"
class="form-control"
id="title"
name="title"
placeholder="Title">
</div>
<span>Details:</span>
<div class="form-group">
<textarea
rows="6"
class="form-control"
name="details">
</textarea>
</div>
<button type="submit" class="btn btn-primary">Add Record</button>
</form>
</div>
</div>
</div>
</div>
@endsection
resourses/views/product_details.blade.php
@extends('layout.app')
@section('main-content')
<div class="row mt-1">
<div class="col-md-8 offset-md-2">
<div class="row">
<div class="col-md-12 text-center">
<h4>Laravel 8 CRUD Tutorial Using Mysql Datatable</h4>
</div>
<div class="col-md-12 mt-1 mr-1">
<div class="float-right">
<a class="btn btn-primary" href="{{ route('product.index') }}"> Back</a>
</div>
</div>
<br><br>
<div class="col-md-12">
<h1>{{$product->title}}</h1>
</div>
<div class="col-md-12">
<p>{{$product->details}}</p>
</div>
</div>
</div>
</div>
@endsection
update product record views file
resourses/views/edit_product.blade.php
@extends('layout.app')
@section('main-content')
<div class="row">
<div class="col-md-8 offset-md-2">
<div class="row">
<div class="col-md-12 text-center">
<h4>Laravel 8 Ajax CRUD Tutorial Using Mysql Datatable</h4>
</div>
<div class="col-md-12 mt-1 mr-1">
<div class="float-right">
<a class="btn btn-primary" href="{{ route('product.index') }}"> Back</a>
</div>
</div>
<div class="col-md-12">
@if ($errors->any())
<div class="alert alert-danger">
<strong>Whoops!</strong> There were some problems with your input.<br><br>
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
</div>
<div class="col-md-12">
<form method="post" action="{{route('product.update',$product->id)}}">
@csrf
@method('PUT')
<div class="form-group">
<label for="title">Title:</label>
<input
type="text"
class="form-control"
id="title"
name="title"
value="{{$product->title}}">
</div>
<div class="form-group">
<label for="details">Details:</label>
<textarea
rows="6"
class="form-control"
name="details">
{{$product->details}}
</textarea>
<button type="submit" class="btn btn-primary">Update Record</button>
</form>
</div>
</div>
</div>
</div>
@endsection
Done, final output
add a new record
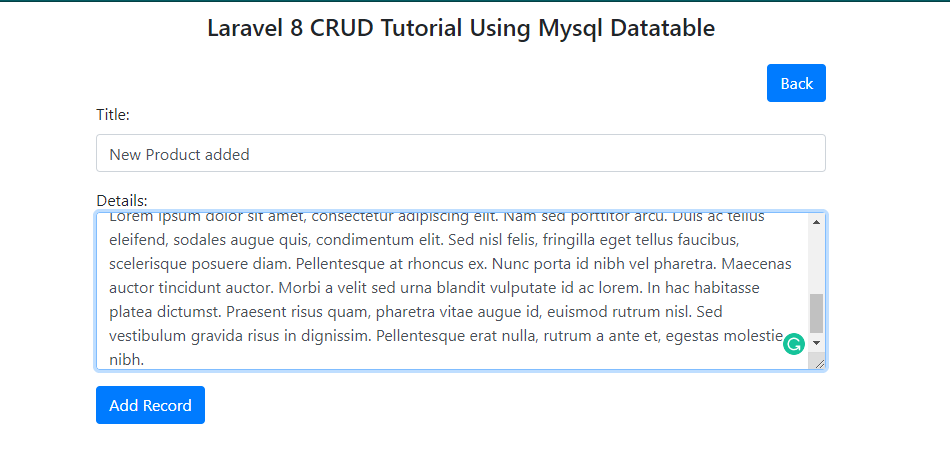
After added mutiple records,
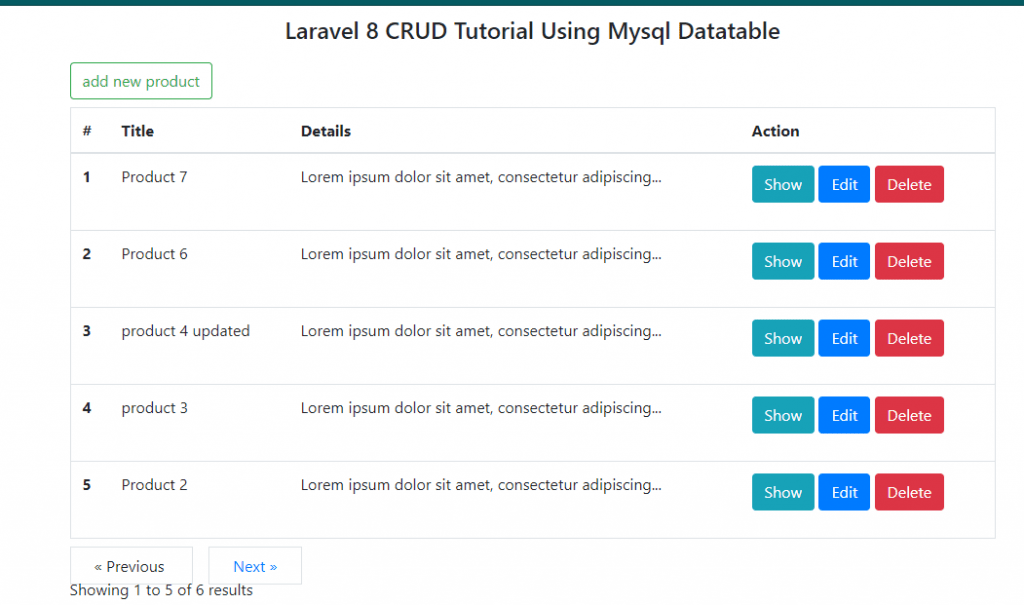
Edit a record
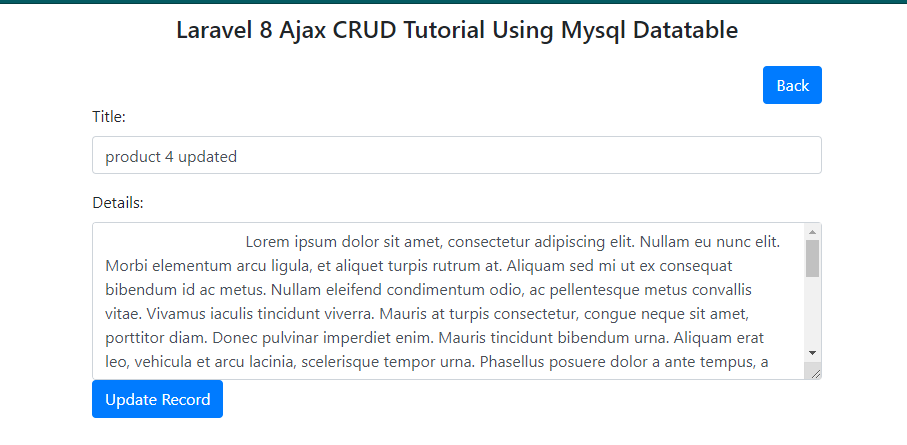
view single record
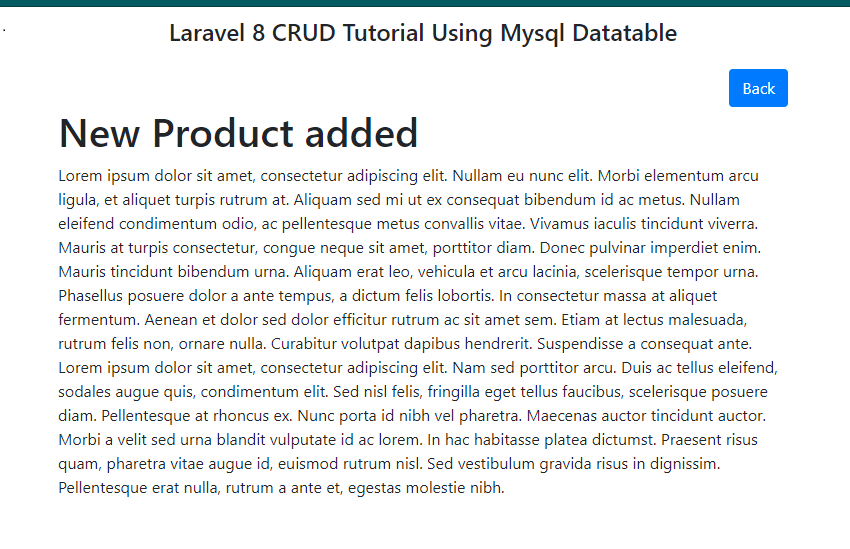
I think, it will help you!
Comments are closed