In this example, we will see how the resource router works. Before that, we will show how normal routes work for the normal crud app.
Resource Routing in Laravel 8.0 makes developers for writing code efficiently and manageable
routes/web.php
Normal crud app routes like this:
use App\Http\Controllers\PostController;
Route::get('/posts', '[PostController::class, 'index']');
Route::get('/posts/create', '[PostController::class, 'create']');
Route::post('/posts', '[PostController::class, 'store']');
Route::get('/posts/{id}/edit', '[PostController::class, 'edit']');
Route::put('/posts/{id}', '[PostController::class, 'update']');
Route::get('/posts/{id}', '[PostController::class, 'show']');
Route::delete('/posts/{id}', '[PostController::class, 'destroy']');
The above routes are responsible for Post managebale functionalities.
Now we want to replace those routes with resource routers.
Read Also
Create Resource Route
php artisan make:controller PostController --resource
And route in the web.php file
routes/web.php
use App\Http\Controllers\PostController; // import at the top
Route::resource('/post',PostController::class);
And the controller should like the below:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class PostController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
//
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
You can check the resources route by running bellow command:
php artisan route:list
It shows all routes
But Specifically want to show route like
php artisan route:list --name=post
output:
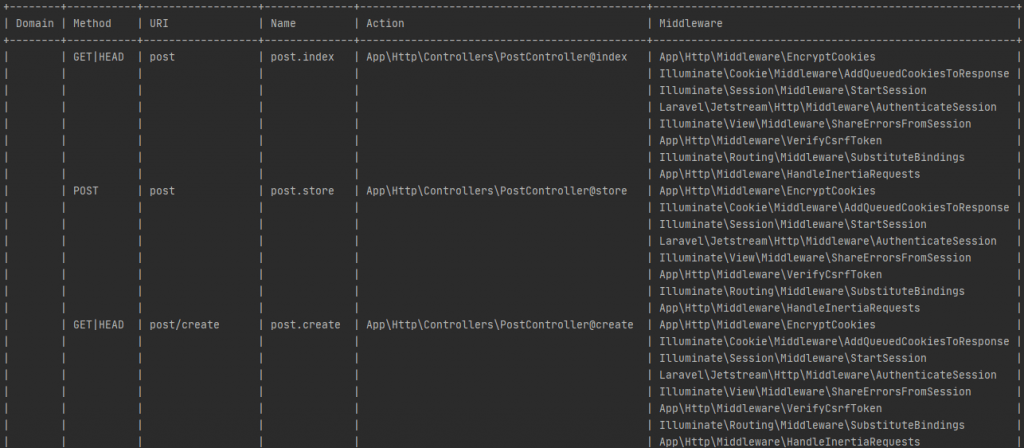
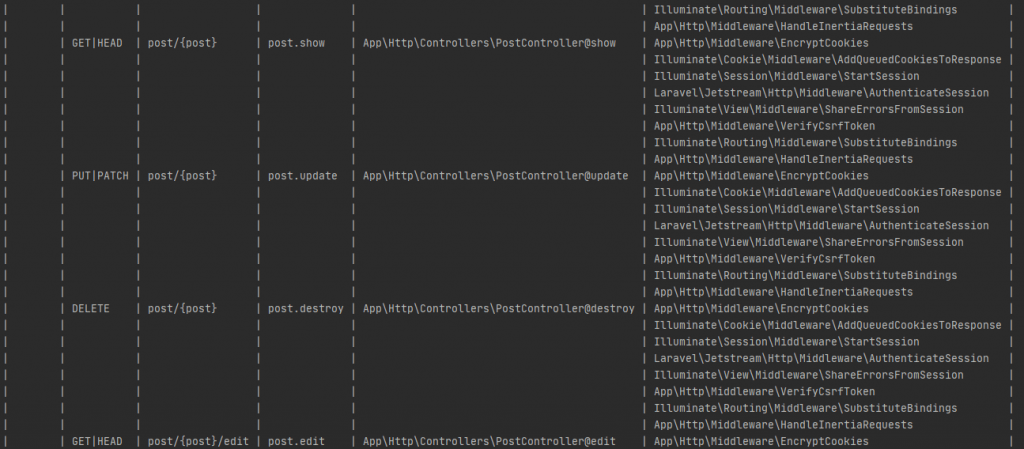
Hope it will help
Comments are closed