Hello artisan, Today I am going to show you an interesting topic. Sometimes you want to display previous and next posts in your blog. Laravel makes it very easy to implement this logic. In this post, you will learn, How to Get the Next and Previous Post in laravel.
Read also
Create a new Laravel 8.0 project
Laravel 8.0 CRUD Tutorial Using Mysql Database
Output looks like
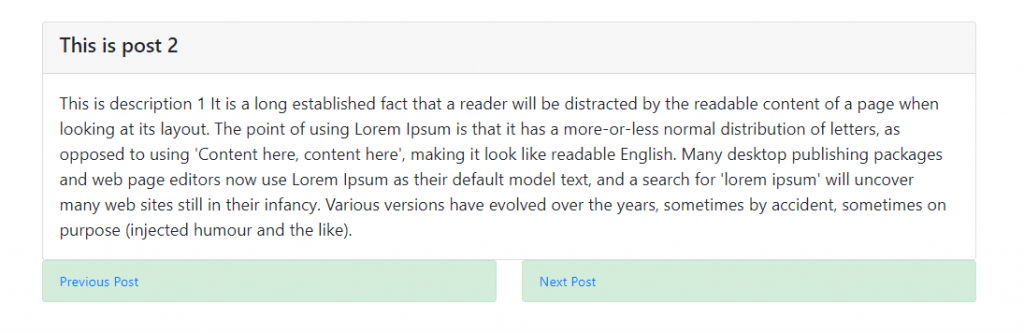
Get Previous Record
$previous_record = Post::where('id', '<', $post->id)->orderBy('id','desc')->first();
Get Next Record
$next_record = Post::where('id', '>', $post->id)->orderBy('id')->first();
Update Controller
app\Http\Controllers\PostController.php
public function show($id)
{
$post = Post::findOrFail($id);
$previous_record = Post::where('id', '<', $post->id)->orderBy('id','desc')->first();
$next_record = Post::where('id', '>', $post->id)->orderBy('id')->first();
return view('single_post',compact('post','previous_record','next_record'));
}
The above code is responsible for fetching a single record from the posts table using id. and also we can find the previous and next records just by writing two lines of code. we send back every record to the single_post.blade.php file.
Update View file
app\resources\views\single_post.blade.php
<div class="container mt-5">
<div class="row">
<div class="col-md-12">
<div class="card">
<div class="card-header">
<h4>{{$post->title}}</h4>
</div>
<div class="card-body">
<blockquote class="blockquote mb-0">
{{$post->body}}
</blockquote>
</div>
</div>
</div>
</div>
<div class="row">
<div class="col-md-6">
@if (isset($previous_record))
<div class="alert alert-success">
<a href="{{ route('posts.show',$previous_record->id) }}">
<div class="btn-content">
<div class="btn-content-title"><i class="fa fa-arrow-left"></i> Previous Post</div>
</div>
</a>
</div>
@endif
</div>
<div class="col-md-6">
@if (isset($next_record))
<div class="alert alert-success">
<a href="{{ route('posts.show',$next_record->id) }}">
<div class="btn-content">
<div class="btn-content-title">Next Post <i class="fa fa-arrow-right"></i></div>
</div>
</a>
</div>
@endif
</div>
</div>
Add routes
app\routes\web.php
Route::resource('/posts',PostController::class);
notice that we use resource routes for our PostController. If you have not then you can create manually.
Output
http://127.0.0.1:8000/posts/
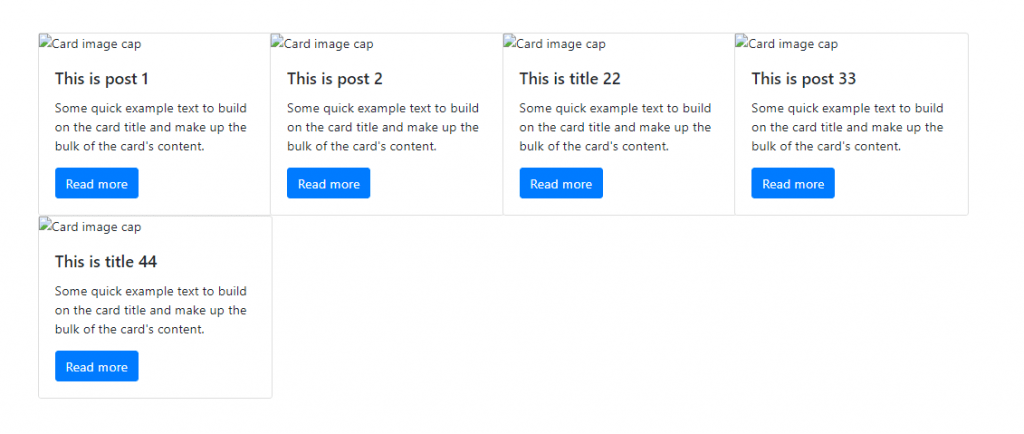
http://127.0.0.1:8000/posts/2
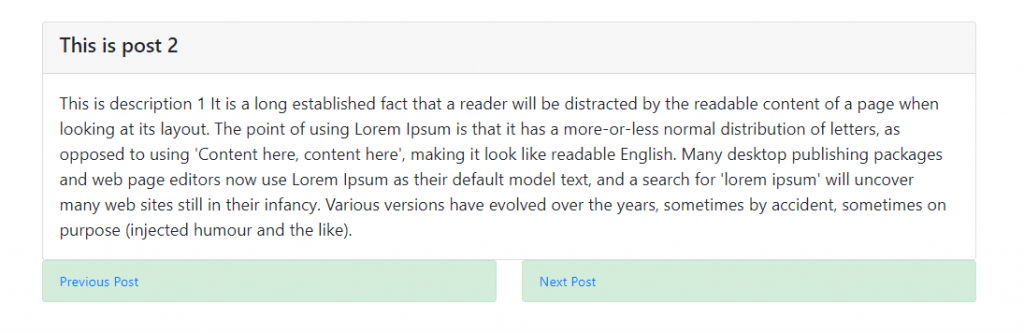
Hope, it will helps!