Hello,
Today we will create a spring boot CRUD application. In, this example, we are using the MySQL database to store data, to view and manage the data we will use react Js library in the frontend part. For API testing we will use Postman.
In this article, We will save data using react application, To make an HTTP request, in this project we will use the Axios library. Actually, in this example, we will save employee data using react UI. Before we saved data using postman.
To make any HTTP post or get a request, we need something that will do for us< for this I am going to use the Axios library. To install the Axios library run the following command.
npm install axios
Create Service Folder:
Firstly create a service folder in the src folder. In this service folder, create an EmployeeService.js file. Basically, in this file, we will keep the Axios-based configuration. For making any post request, I will create a save method that takes the URL and data and returns the response.
src => service => EmployeeService.js
import axios from "axios";
const baseUrl = "http://localhost:8090/api/v1"
var config = {
method: 'post',
headers: {
'Content-Type': 'application/json'
}
};
export const save = (url, data) => {
return axios({ ...config, url: baseUrl + url, data: data })
}
Go to the AddEmployees.js file
import React, { useState } from 'react'
import { Button, Alert, Container, Row, Card, Col, Form, InputGroup } from 'react-bootstrap'
import { save } from '../service/EmployeeService'
const AddEmployees = () => {
const [status, setStatus] = useState("")
const [employee, setEmployee] = useState({
"firstName": "",
"lastName": "",
"email": "",
"address": "",
"designation": "",
"age": "",
})
const addEmployeeHandler = (e) => {
e.preventDefault()
save("/employees", employee)
.then(res => {
setStatus(res.data)
})
.catch(err => {
setStatus(err)
})
}
return (
<>
<Container>
<Row>
<Col></Col>
<Col>
<Card>
<Card.Header className='text-center'>
Add New Employee
</Card.Header>
<Card.Body>
{status.length > 0 && (
<Alert variant='success'>{status}</Alert>
)}
<Form onSubmit={addEmployeeHandler}>
<InputGroup className="mb-3">
<InputGroup.Text id="basic-addon3">
First Name
</InputGroup.Text>
<Form.Control id="basic-url" aria-describedby="basic-addon3"
onChange={e => {
setEmployee({
...employee,
firstName: e.target.value
})
}} />
</InputGroup>
<InputGroup className="mb-3">
<InputGroup.Text id="basic-addon3">
Last Name
</InputGroup.Text>
<Form.Control id="basic-url" aria-describedby="basic-addon3"
onChange={e => {
setEmployee({
...employee,
lastName: e.target.value
})
}}
/>
</InputGroup>
<InputGroup className="mb-3">
<InputGroup.Text id="basic-addon3">
Email
</InputGroup.Text>
<Form.Control id="basic-url" aria-describedby="basic-addon3"
onChange={e => {
setEmployee({
...employee,
email: e.target.value
})
}}
/>
</InputGroup>
<InputGroup className="mb-3">
<InputGroup.Text id="basic-addon3">
Address
</InputGroup.Text>
<Form.Control id="basic-url" aria-describedby="basic-addon3"
onChange={e => {
setEmployee({
...employee,
address: e.target.value
})
}}
/>
</InputGroup>
<InputGroup className="mb-3">
<InputGroup.Text id="basic-addon3">
Designation
</InputGroup.Text>
<Form.Control id="basic-url" aria-describedby="basic-addon3"
onChange={e => {
setEmployee({
...employee,
designation: e.target.value
})
}}
/>
</InputGroup>
<InputGroup className="mb-3">
<InputGroup.Text id="basic-addon3">
Age
</InputGroup.Text>
<Form.Control id="basic-url" aria-describedby="basic-addon3"
onChange={e => {
setEmployee({
...employee,
age: e.target.value
})
}}
type='number' />
</InputGroup>
<InputGroup className='text-center'>
<Button type='submit'>Create Employee record</Button>
</InputGroup>
</Form>
</Card.Body>
</Card>
</Col>
<Col></Col>
</Row>
</Container>
</>
)
}
export default AddEmployees
Firstly, import the save method from the EmployeeService.js file.
Then, we created an employee state, and using the onChange() method we assigned each and every value to the employee object.
Then created an addEmployeeHandler method which will mainly use the save method and be responsible for making the HTTP post request. and after the request, if it is a success then we kept the success response to the status object using the setStatus method.
Before making any request, we have to change the backend just look like this:
@PostMapping("/employees")
public ResponseEntity<?> createEmployee(@RequestBody Employee employee) {
employeeService.save(employee);
return new ResponseEntity<>("Employee Created Successfully",HttpStatus.CREATED);
}
If you faced any cors-origin errors, then add the below code in the controller class definition.
@CrossOrigin(origins = "http://localhost:3000/")
The final version of the EmployeeController.java class is:
package com.crud.controller;
import com.crud.entity.Employee;
import com.crud.service.EmployeeService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
@CrossOrigin(origins = "http://localhost:3000/")
@RestController
@RequestMapping("/api/v1")
public class EmployeeController {
@Autowired
private EmployeeService employeeService;
@PostMapping("/employees")
public ResponseEntity<?> createEmployee(@RequestBody Employee employee) {
employeeService.save(employee);
return new ResponseEntity<>("Employee Created Successfully",HttpStatus.CREATED);
}
}
Alright, run the react application, click the add-employee link and try to save data:
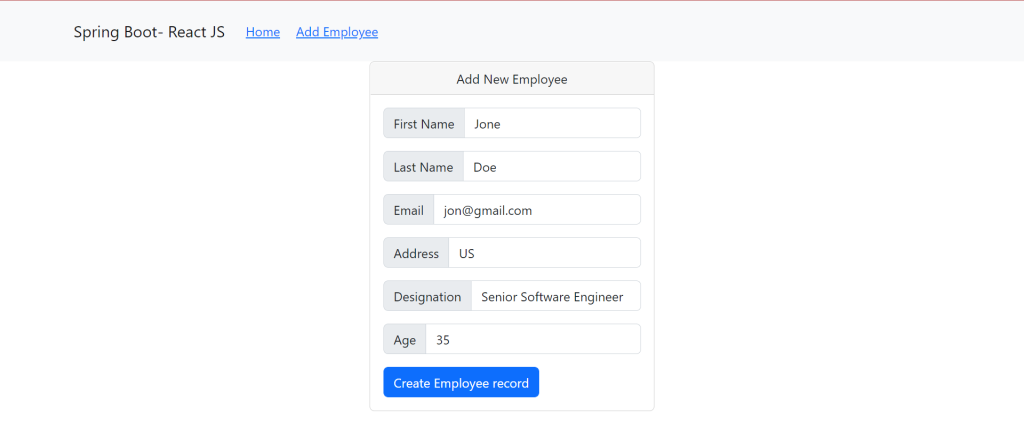
The Response looks like this:
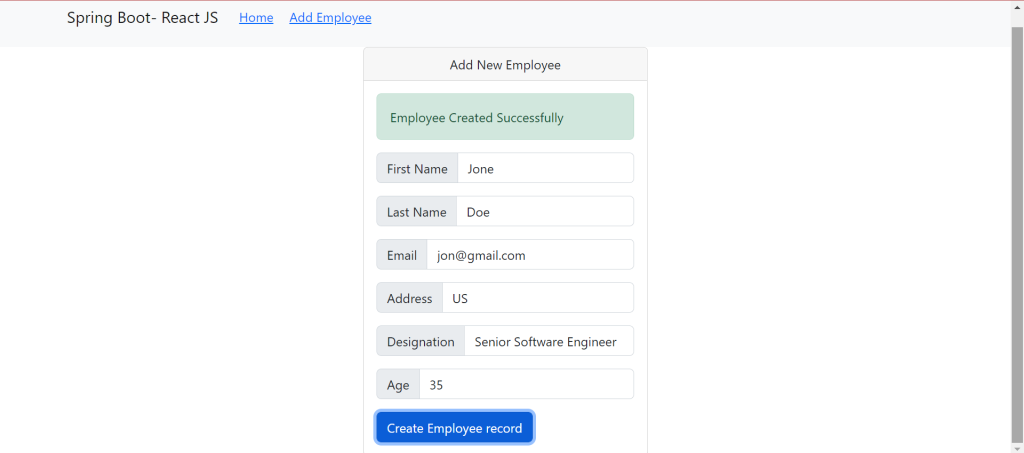
Hope you enjoyed it. If you face any issues please feel free to comment.