Hello friends, Today I am going to show you how to connect your MySQL database with your JSP and servlet web app. In this example, we will connect our JSP and servlet web app with the MySQL database.
To get the MySQL database, we will install xampp application. In xampp we can get the features of MySQL database and apache server to visualize the MySQL data.
Download xampp from here. You can read the below article on how to install the xampp.
How to install xampp in windows 10 | PHP (codesnipeet.com)
Create a web app
File => New => Dynamic Web Project
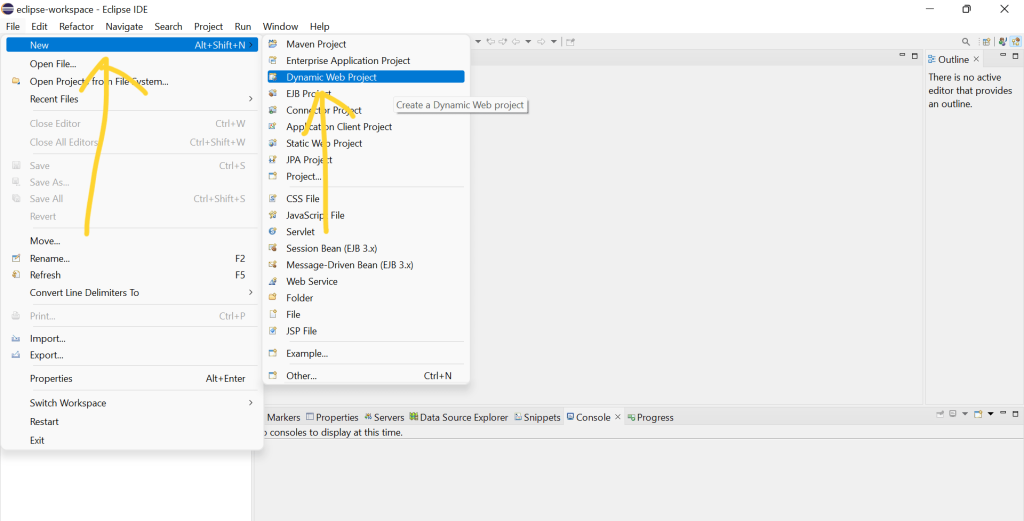
Give any name => Finish
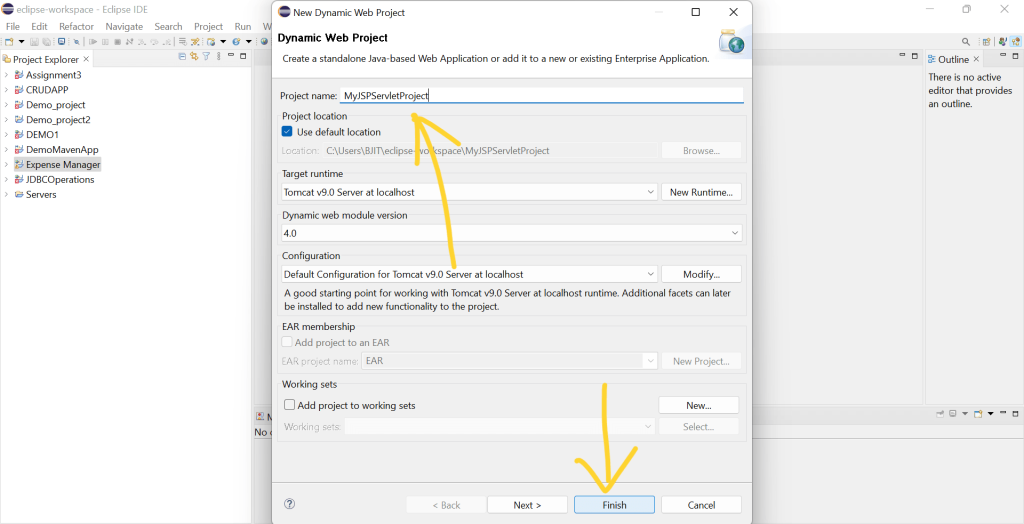
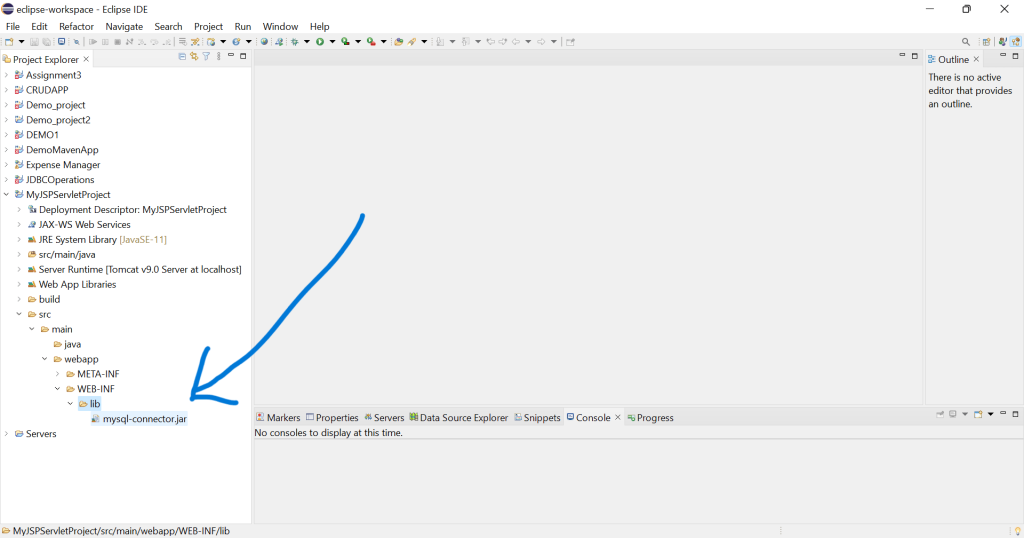
Download the MySQL connector jar. This is must be required to connect MySQL database. Download from here.
Copy the jar file and paste it into the lib folder.
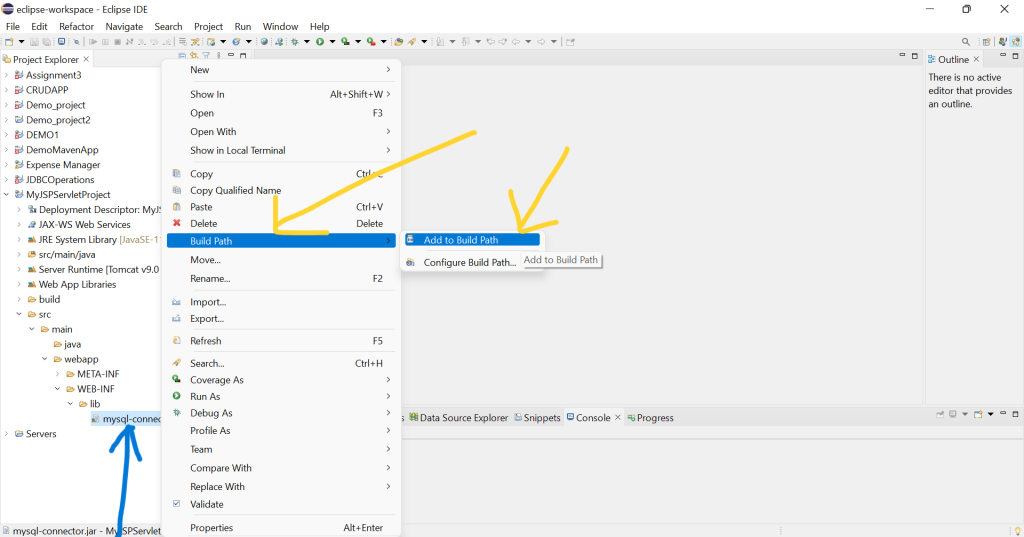
Right-click on the jar file then go to Build Path => Add to Build Path
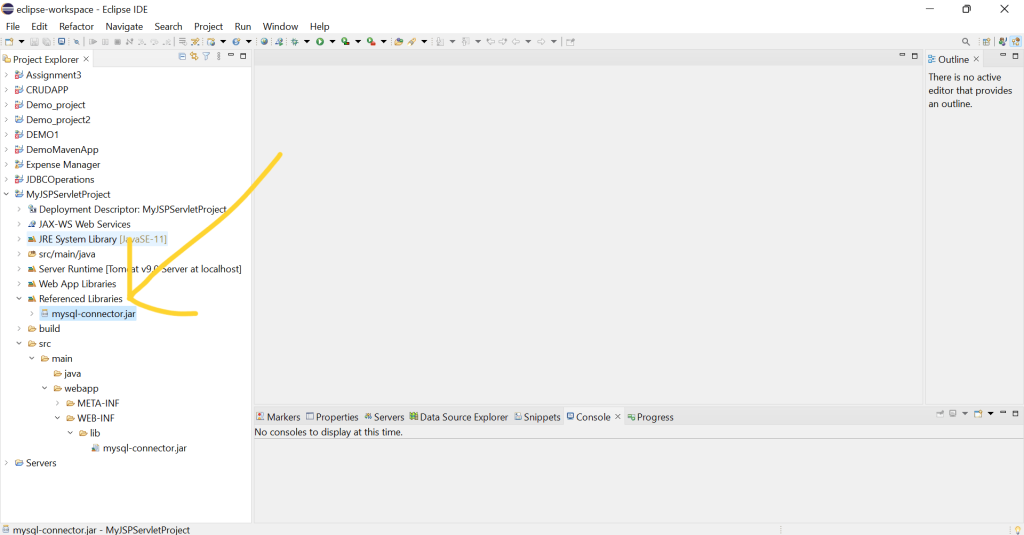
The jar was added successfully. Then write code to connect the database.
Create a class JDBCDao.java under com.dao package, and write down the below code.
package com.jdbc;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class JDBCDao {
static final String DB_URL = "jdbc:mysql://localhost:3306/expense_management_system";
static final String USER = "root";
static final String PASS = "";
static final String JDBCDRIVER = "com.mysql.jdbc.Driver";
public void loadDriver(String driver) {
try {
Class.forName(driver);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public Connection getDatabaseConnection() {
loadDriver(JDBCDRIVER);
Connection conn = null;
try {
System.out.println("Connecting to the database...");
conn = DriverManager.getConnection(DB_URL, USER, PASS);
System.out.println("Connected!");
} catch (SQLException se) {
se.printStackTrace();
}
return conn;
}
public static void main(String[] args) {
JDBCDao dao = new JDBCDao();
dao.getDatabaseConnection();
}
}
To check the connection whether is connected or not, create a main() method and within the main() method create an instance of JDBCDao class and call the getConnection() method.
Run the file:
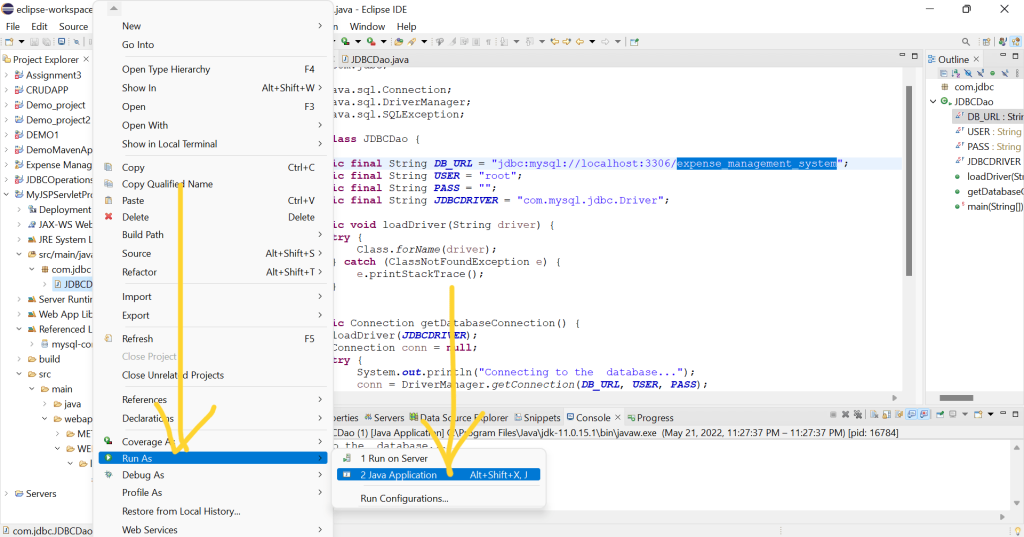
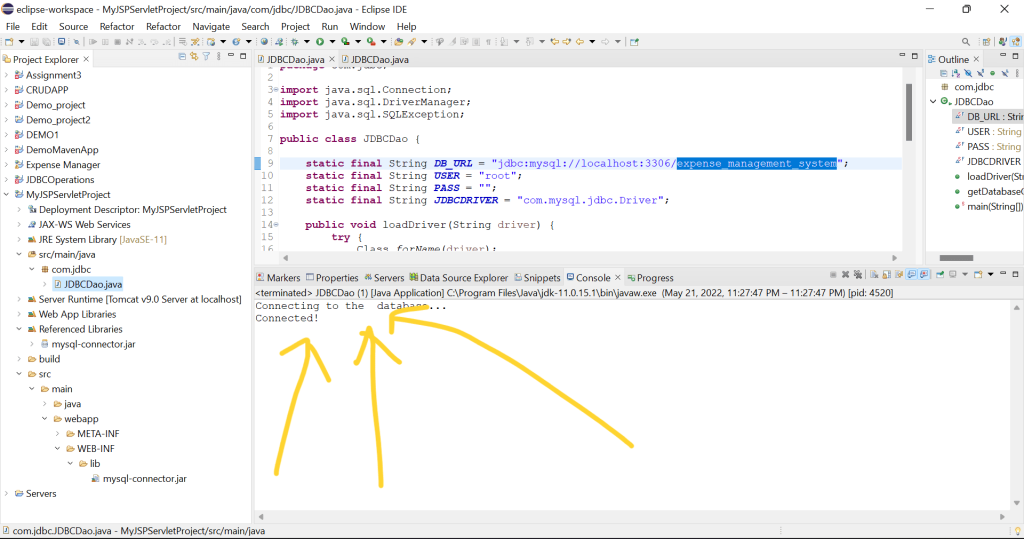
Wow, the MySQL Database is connected. If face any problems please comment.