Hello,
Today we will create a spring boot CRUD application. In, this example, we are using the MySQL database to store data, to view and manage the data we will use react Js library in the frontend part. For API testing we will use Postman.
In this article, We will delete the employee record from the database. Today, we will remove the employee records by their employee id.
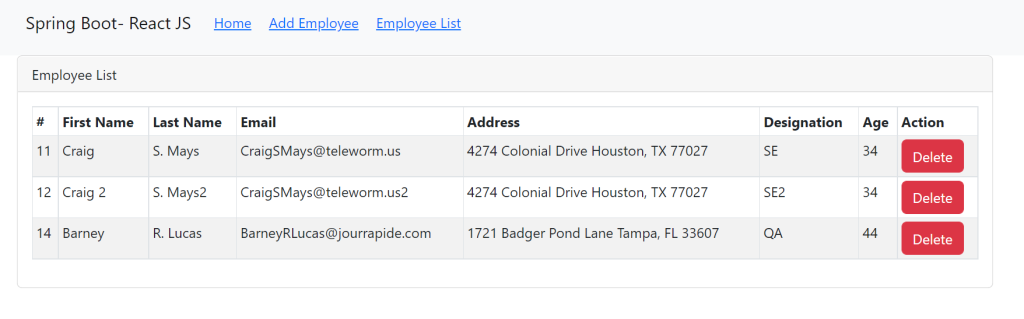
After Deleting…
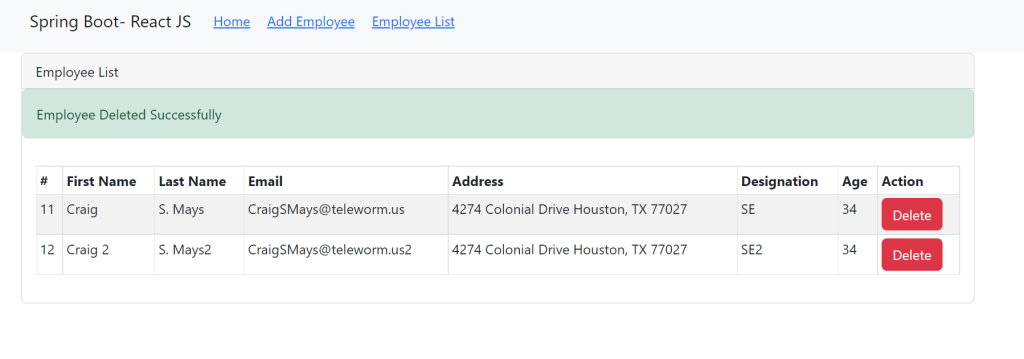
So, let’s start. Firstly we have to create the delete mapping method. Using this method, we can remove the employee record by their employee id.
So, go to the EmployeeController class and this below method.
@DeleteMapping("/employees/{employee-id}")
public ResponseEntity<?> deleteEmployeeById(@PathVariable("employee-id") long employeeId){
employeeService.deleteEmployeeById(employeeId);
return new ResponseEntity<>("Employee Deleted Successfully",HttpStatus.OK);
}
Add the deleteEmployeeById method in the EmployeeService Interface.
void deleteEmployeeById(long employeeId);
Add the implementation of the deleteEmployeeById method in the EmployeeServiceImpl class.
@Override
public void deleteEmployeeById(long employeeId) {
employeeRepository.deleteById(employeeId);
}
So the final version of the EmployeeController class is:
package com.crud.controller;
import com.crud.entity.Employee;
import com.crud.service.EmployeeService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@CrossOrigin(origins = "http://localhost:3000/")
@RestController
@RequestMapping("/api/v1")
public class EmployeeController {
@Autowired
private EmployeeService employeeService;
@PostMapping("/employees")
public ResponseEntity<?> createEmployee(@RequestBody Employee employee) {
employeeService.save(employee);
return new ResponseEntity<>("Employee Created Successfully",HttpStatus.CREATED);
}
@GetMapping("/employees")
public ResponseEntity<List<Employee>> getEmployees(){
return ResponseEntity.ok(employeeService.getEmployees());
}
@DeleteMapping("/employees/{employee-id}")
public ResponseEntity<?> deleteEmployeeById(@PathVariable("employee-id") long employeeId){
employeeService.deleteEmployeeById(employeeId);
return new ResponseEntity<>("Employee Deleted Successfully",HttpStatus.OK);
}
}
The final version of the EmployeeService interface is:
package com.crud.service;
import com.crud.entity.Employee;
import java.util.List;
public interface EmployeeService {
Employee save(Employee employee);
List<Employee> getEmployees();
void deleteEmployeeById(long employeeId);
}
And the final version of the EmployeeServiceImpl class is:
package com.crud.serviceImpl;
import com.crud.entity.Employee;
import com.crud.repository.EmployeeRepository;
import com.crud.service.EmployeeService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class EmployeeServiceImpl implements EmployeeService {
@Autowired
private EmployeeRepository employeeRepository;
@Override
public Employee save(Employee employee) {
return employeeRepository.save(employee);
}
@Override
public List<Employee> getEmployees() {
return employeeRepository.findAll();
}
@Override
public void deleteEmployeeById(long employeeId) {
employeeRepository.deleteById(employeeId);
}
}
Now go to the frontend part. Create a remove method in the EmployeeService.js file.
Declare the config first:
var deleteConfig = {
method: 'delete',
headers: {}
};
Create the remove method:
export const remove = (url) => {
return axios({ ...deleteConfig, url: baseUrl + url })
}
The Final version of the EmployeeService.js file is:
import axios from "axios";
const baseUrl = "http://localhost:8090/api/v1"
var postConfig = {
method: 'post',
headers: {
'Content-Type': 'application/json'
}
}
var getConfig = {
method: 'get',
headers: {}
};
var deleteConfig = {
method: 'delete',
headers: {}
};
export const save = (url, data) => {
return axios({ ...postConfig, url: baseUrl + url, data: data })
}
export const get = (url) => {
return axios({ ...getConfig, url: baseUrl + url })
}
export const remove = (url) => {
return axios({ ...deleteConfig, url: baseUrl + url })
}
Now come to the Employees.js file. and add another th and td element in the previous table. In the td element set an onClick handler method and declare the method which will take the employee ID as an argument. and call the remove method from the EmployeeService.js file.
------
<th>Action</th>
------
<td>
<Button className='btn btn-danger' onClick={() => removeHandler(employee.id)}> Delete</Button>
</td>
Create the onClick method:
const removeHandler = (id) => {
remove("/employees/" + id)
.then(res => {
setStatus(res.data)
getEmployees()
})
.catch(err => console.log(err))
}
In this method, we will first call the delete mapping method. If the method return success then we will again call the getEmployees() method to re-render the employeeList. And also set the success status state which will be printed using Alert.
The final version of the Employees.js file is:
import React, { useEffect, useState } from 'react'
import { Container, Row, Col, Card, Table, Button, Alert } from 'react-bootstrap'
import { get, remove } from '../service/EmployeeService'
const Employees = () => {
const [employees, setEmployees] = useState([])
const [status, setStatus] = useState("")
const getEmployees = () => {
get("/employees")
.then(res => {
console.log(res.data)
setEmployees(res.data)
})
.catch(err => console.log(err))
}
useEffect(() => {
getEmployees() // called the method each time when the page is loaded
}, [])
const removeHandler = (id) => {
remove("/employees/" + id)
.then(res => {
setStatus(res.data)
getEmployees()
})
.catch(err => console.log(err))
}
return (
<>
<Container>
<Row>
<Col>
<Card>
<Card.Header>Employee List</Card.Header>
{status.length > 0 && (<Alert variant='success'>{status}</Alert>)}
<Card.Body>
<Table striped bordered hover size="sm">
<thead>
<tr>
<th>#</th>
<th>First Name</th>
<th>Last Name</th>
<th>Email</th>
<th>Address</th>
<th>Designation</th>
<th>Age</th>
<th>Action</th>
</tr>
</thead>
<tbody>
{
employees?.map(employee => {
return (
<tr key={employee.id}>
<td>{employee.id}</td>
<td>{employee.firstName}</td>
<td>{employee.lastName}</td>
<td>{employee.email}</td>
<td>{employee.address}</td>
<td>{employee.designation}</td>
<td>{employee.age}</td>
<td>
<Button className='btn btn-danger' onClick={() => removeHandler(employee.id)}> Delete</Button>
</td>
</tr>
)
})
}
</tbody>
</Table>
</Card.Body>
</Card>
</Col>
</Row>
</Container>
</>
)
}
export default Employees
Hope it helps
Output:
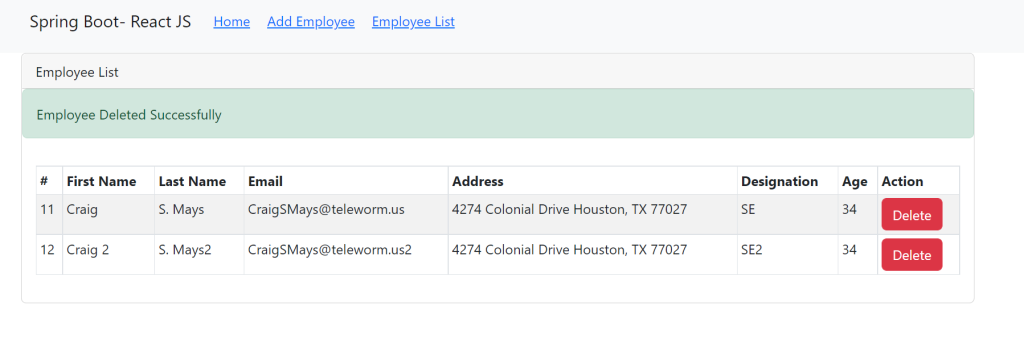